Create invoices by writing text in PDF templates in PHP
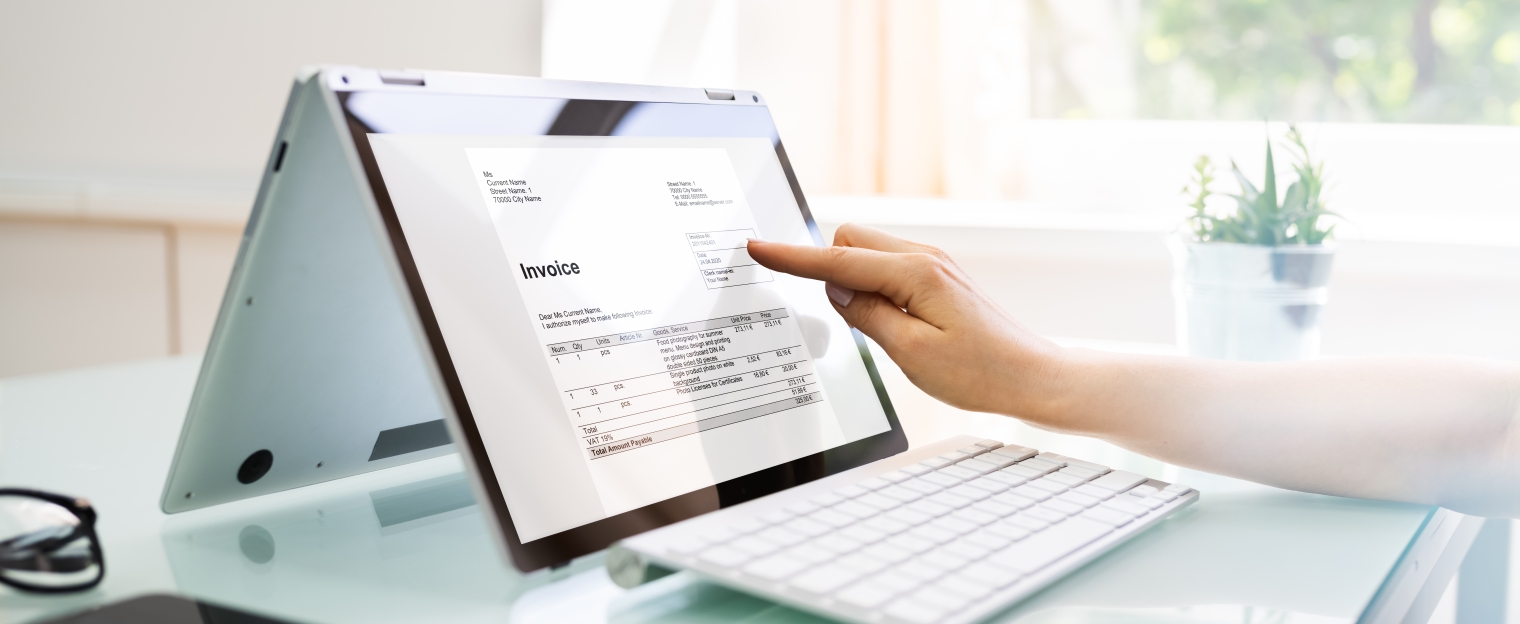
Introduction.
I would like to create an invoice by placing names, addresses, etc. on a PDF template.
Use FPDI to read the template PDF.
Use TCPDF to place text on the read template PDF.
Use FPDI to read the template PDF.
Use TCPDF to place text on the read template PDF.
Install TCPDF and FPDI
First, install TCPDF and FPDI with Composer.
composer install
composer install
composer.json
{
"require": {
"setasign/fpdi": "^2.3",
"tecnickcom/tcpdf": "^6.4"
}
}
PHP Code
In line 23 and following, the position of the address 2 and the company name is adjusted in case they are missing.
In addition, if there is no name, the company name is preprocessed by adding "御中" to the name of the company.
The following will export the generated PDF file to the data folder in the same hierarchy.
$pdf->Output($file, 'F');
The template was created in Adobe Illustrator.
Below is the full code.
In addition, if there is no name, the company name is preprocessed by adding "御中" to the name of the company.
The following will export the generated PDF file to the data folder in the same hierarchy.
$pdf->Output($file, 'F');
The template was created in Adobe Illustrator.
Below is the full code.
PHP
<?php
require_once __DIR__ . '/vendor/tecnickcom/tcpdf/tcpdf.php';
require_once __DIR__ . '/vendor/setasign/fpdi/src/autoload.php';
use setasign\Fpdi\Tcpdf\Fpdi;
$invoice_number = 1;
$file = __DIR__ . '/data/' . $invoice_number . '.pdf';
$template = __DIR__ . '/template.pdf';
$data = [
'no' => '請求書番号: ' . $invoice_number,
'date' => '請求書発行日: ' . date('Y年n月j日'),
'user_id' => '会員No: 1',
'company' => '海山商事株式会社',
'name' => '名前太郎様',
'zip' => '〒100-0001',
'address1' => '東京都千代田区',
'address2' => '○○町1-1-1',
'product' => '商品名',
'price' => '¥ 9,800 -'
];
$margin = 7;
if ('' === $data['address2']) {
$position_company = 66;
} else {
$position_company = 73;
}
$position_name = $position_company + $margin;
$position_user_id = $position_name + $margin;
if ('' === $data['company']) {
$position_name -= $margin;
$position_user_id -= $margin;
}
$pdf = new setasign\Fpdi\Tcpdf\Fpdi('P', 'mm', 'A4');
$pdf->SetMargins(0, 0, 20);
$pdf->SetAutoPageBreak(false);
$pdf->setPrintHeader(false);
$pdf->setPrintFooter(false);
$pdf->SetTextColor(0, 0, 0);
$pdf->SetSourceFile($template);
$p1 = $pdf->importPage(1);
$pdf->AddPage();
$pdf->useTemplate($p1, null, null, null, null, true);
// 日付
$pdf->SetFont('kozgopromedium', '', 9);
$pdf->SetXY(0, 43);
$pdf->Write(0, $data['date'], '', FALSE, 'R');
// 請求書No
$pdf->SetFont('kozgopromedium', '', 9);
$pdf->SetXY(0, 50);
$pdf->Write(0, $data['no'], '', FALSE, 'R');
// 住所
$pdf->SetFont('kozgopromedium', '', 10);
$pdf->SetXY(23, 52);
$pdf->Write(0, $data['zip']);
$pdf->SetFont('kozgopromedium', '', 10);
$pdf->SetXY(23, 58);
$pdf->Write(0, $data['address1']);
$pdf->SetFont('kozgopromedium', '', 10);
$pdf->SetXY(23, 64);
$pdf->Write(0, $data['address2']);
// 会社名
$pdf->SetFont('kozgopromedium', '', 12);
$pdf->SetXY(23, $position_company);
$pdf->Write(0, $data['company']);
// 名前
$pdf->SetFont('kozgopromedium', '', 12);
$pdf->SetXY(23, $position_name);
$pdf->Write(0, $data['name']);
// 会員No
$pdf->SetFont('kozgopromedium', '', 8);
$pdf->SetXY(23, $position_user_id);
$pdf->Write(0, $data['user_id']);
// 商品名
$pdf->SetFont('kozminproregular', '', 12);
$pdf->SetXY(53, 122.5);
$pdf->Write(0, $data['product']);
// 金額
$pdf->SetFont('kozminproregular', '', 22);
$pdf->SetXY(53, 134);
$pdf->Write(0, $data['price']);
$pdf->Output($file, 'F');
finished product
At the end.
When you have to send a large number of invoices on the closing day, you can use cron to automatically generate PDF files and send them as email attachments.
I would also like to change to a font other than the default one, or use a tabular format, but I decided not to do so this time.
I would also like to change to a font other than the default one, or use a tabular format, but I decided not to do so this time.
PROFILE
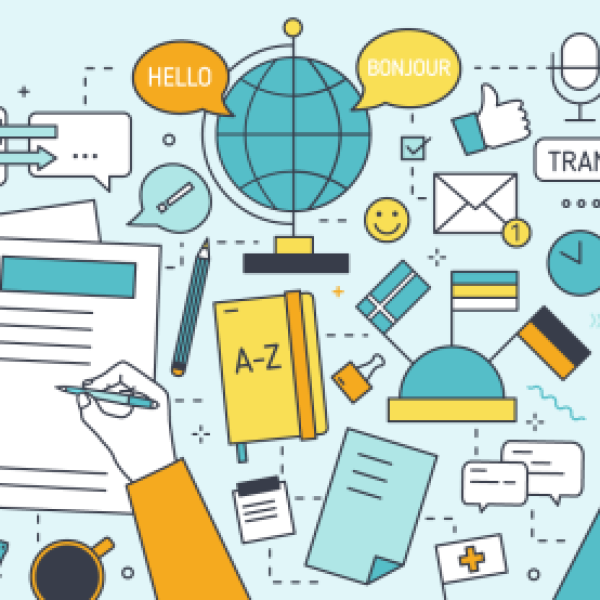
- C:blanc
- I am a freelance programmer.
COMMENT
Submitted. Comments will appear after approval.