PHP generation of invoice issuing data for Yamato Transport's B2 Cloud
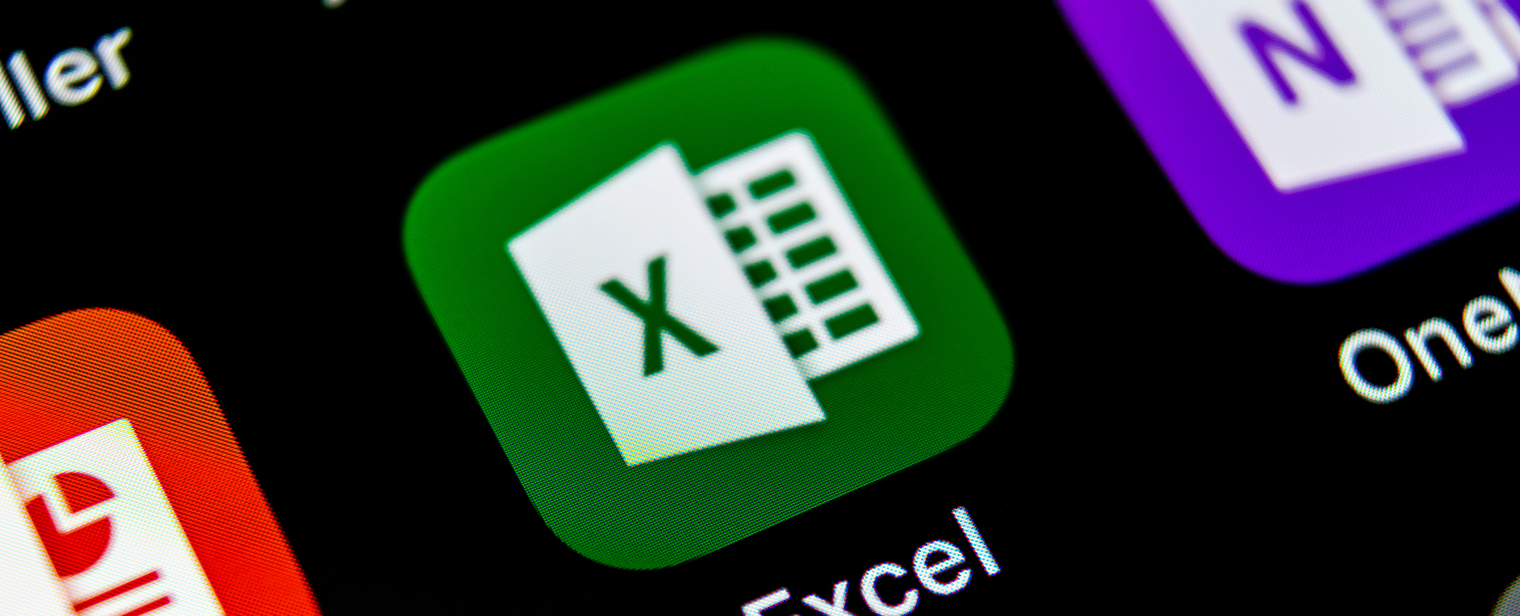
Introduction.
I would like to export an XLSX file of Yamato Transport's B2Cloud invoice issuance data using PhpSpreadsheet .
The specifications are as follows
B2Cloud invoice issuance data layout for input/output (PDF)
The specifications are as follows
B2Cloud invoice issuance data layout for input/output (PDF)
Install PhpSpreadsheet
First, install PhpSpreadsheet with Composer.
composer install
composer install
composer.json
{
"require": {
"phpoffice/phpspreadsheet": "^1.23"
}
}
PHP Code
There are no particular difficulties, but be careful not to mistake lines because there are many items.
The sample does not fill in all items, so if you need more items, add them based on the specifications.
If you have multiple delivery addresses, add data to the $data array.
In practice, you would get the data from a database or other source in a loop.
The sample does not fill in all items, so if you need more items, add them based on the specifications.
If you have multiple delivery addresses, add data to the $data array.
In practice, you would get the data from a database or other source in a loop.
PHP
<?php
$results = [];
$results[] = [
'お客様管理番号',
'送り状種類',
'クール区分',
'伝票番号',
'出荷予定日',
'お届け予定(指定)日',
'配達時間帯',
'お届け先コード',
'お届け先電話番号',
'お届け先電話番号枝番',
'お届け先郵便番号',
'お届け先住所',
'お届け先住所(アパートマンション名)',
'お届け先会社・部門1',
'お届け先会社・部門2',
'お届け先名',
'お届け先名略称カナ',
'敬称',
'ご依頼主コード',
'ご依頼主電話番号',
'ご依頼主電話番号枝番',
'ご依頼主郵便番号',
'ご依頼主住所',
'ご依頼主(アパートマンション名)',
'ご依頼主名',
'ご依頼主略称カナ',
'品名コード1 ',
'品名1',
'品名コード2',
'品名2',
'荷扱い1',
'荷扱い2',
'記事',
'コレクト代金引換額(税込)',
'コレクト内消費税額等',
'営業所止置き',
'営業所コード',
'発行枚数',
'個数口枠の印字',
'ご請求先顧客コード',
'ご請求先分類コード',
'運賃管理番号',
'クロネコwebコレクトデータ登録',
'クロネコwebコレクト加盟店番号',
'クロネコwebコレクト申込受付番号1',
'クロネコwebコレクト申込受付番号2',
'クロネコwebコレクト申込受付番号3',
'お届け予定eメール利用区分',
'お届け予定eメールe-mailアドレス',
'入力機種',
'お届け予定eメールメッセージ',
'お届け完了eメール利用区分',
'お届け完了eメールe-mailアドレス',
'お届け完了eメールメッセージ',
'クロネコ収納代行利用区分',
'予備',
'収納代行請求金額(税込)',
'収納代行内消費税額等',
'収納代行請求先郵便番号',
'収納代行請求先住所',
'収納代行請求先住所(アパートマンション名)',
'収納代行請求先会社・部門名1',
'収納代行請求先会社・部門名2',
'収納代行請求先名(漢字)',
'収納代行請求先名(カナ)',
'収納代行問合せ先名(漢字)',
'収納代行問合せ先郵便番号',
'収納代行問合せ先住所',
'収納代行問合せ先住所(アパートマンション名)',
'収納代行問合せ先電話番号',
'収納代行管理番号',
'収納代行品名',
'収納代行備考',
'複数口くくりキー',
'検索キータイトル1',
'検索キー1',
'検索キータイトル2',
'検索キー2',
'検索キータイトル3',
'検索キー3',
'検索キータイトル4',
'検索キー4',
'検索キータイトル5',
'検索キー5',
'予備',
'予備',
'投函予定メール利用区分',
'投函予定メールe-mailアドレス',
'投函予定メールメッセージ',
'投函完了メール(お届け先宛)利用区分',
'投函完了メール(お届け先宛)e-mailアドレス',
'投函完了メール(お届け先宛)メールメッセージ',
'投函完了メール(ご依頼主宛)利用区分',
'投函完了メール(ご依頼主宛)e-mailアドレス',
'投函完了メール(ご依頼主宛)メールメッセージ',
'連携管理番号',
'通知メールアドレス'
];
$file_name = 'b2.xlsx';
$product_no = '商品番号001';
$product_name = '商品名';
$data = [];
$data[] = '1'; // A:オーダーNo
$data[] = '0';
$data[] = '0';
$data[] = '';
$data[] = '2022/07/13';
$data[] = '最短日';
$data[] = '0812';
$data[] = '';
$data[] = '03-0000-0002';
$data[] = ''; // J
$data[] = '100-0001'; // K
$data[] = '東京都千代田区'; // L
$data[] = '○○町1-1-1'; // M
$data[] = '';
$data[] = '';
$data[] = '名前太郎'; // P
$data[] = '';
$data[] = '様'; // R
$data[] = '1'; // S:依頼主コード
$data[] = '03-0000-0001'; // T:ご依頼主電話番号
$data[] = '';
$data[] = '150-0041';
$data[] = '東京都渋谷区○○1-1-1';
$data[] = '○○ビル1F';
$data[] = '○○株式会社';
$data[] = ''; // Z
$data[] = substr($product_no, 0, 30); // AA:品名コード1
$data[] = mb_substr($product_name, 0, 25); // AB:品名1
$data[] = '';
$data[] = '';
$data[] = 'ナマモノ'; // AE
$data[] = '';
$data[] = '記事'; // AG:記事
$data[] = '3800'; // AH
$data[] = ''; // AI
$data[] = '0'; // AJ
$data[] = '';
$data[] = '1'; // AL:発行枚数
$data[] = '3'; // AM:個数口表示フラグ
$data[] = '0311111111'; // AN:請求先顧客コード
$data[] = '';
$data[] = '01'; // AP:運賃管理番号
$data[] = '0'; // AQ
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = ''; // AU
$data[] = '1'; // AV:お届け予定eメール利用区分
$data[] = 'user@mail.com'; // AW
$data[] = '1'; // AX
$data[] = '○○株式会社オンラインストアでご注文頂いた商品を本日クロネコヤマトで発送致しました。'; // AY
$data[] = '0'; // AZ
$data[] = ''; // BA
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = ''; // CA
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$data[] = '';
$results[] = $data;
succession
PHP
require_once __DIR__ . '/vendor/autoload.php';
use PhpOffice\PhpSpreadsheet\Spreadsheet;
use PhpOffice\PhpSpreadsheet\Writer\Xlsx;
$spreadsheet = new Spreadsheet();
$sheet = $spreadsheet->getActiveSheet();
$sheet->fromArray($results, NULL, 'A1', TRUE);
$writer = new Xlsx($spreadsheet);
header('Content-Description: File Transfer');
header('Content-Disposition: attachment; filename="' . $file_name . '"');
header('Content-Type: application/vnd.openxmlformats-officedocument.spreadsheetml.sheet');
header('Content-Transfer-Encoding: binary');
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Expires: 0');
ob_end_clean();
$writer->save('php://output');
Excel file to be downloaded
At the end.
If you want to use it in a local environment, you will need docker, etc. (For mac, you can use Sites.) (For mac, Sites can be used.)
The PHP code data is a sample. Please do not use it as is. (Please do not use it as is.)
Please rewrite everything based on the specifications.
The PHP code data is a sample. Please do not use it as is. (Please do not use it as is.)
Please rewrite everything based on the specifications.
PROFILE
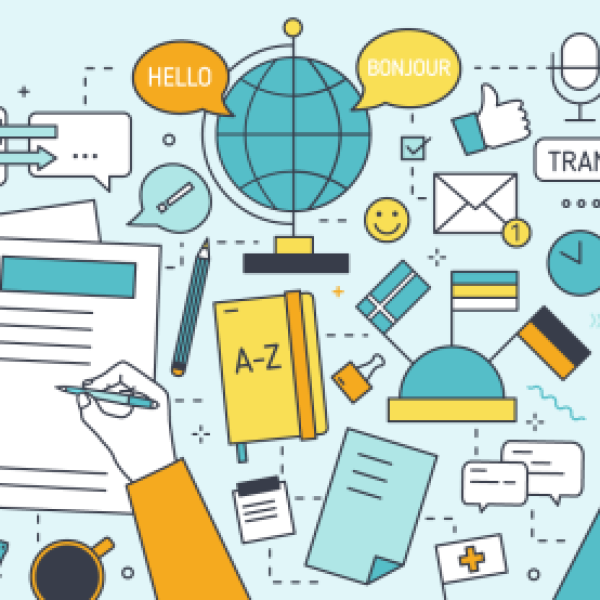
- C:blanc
- I am a freelance programmer.
COMMENT
Submitted. Comments will appear after approval.